September 8, 2023 - 7 min
Laravel Vapor — Getting Started
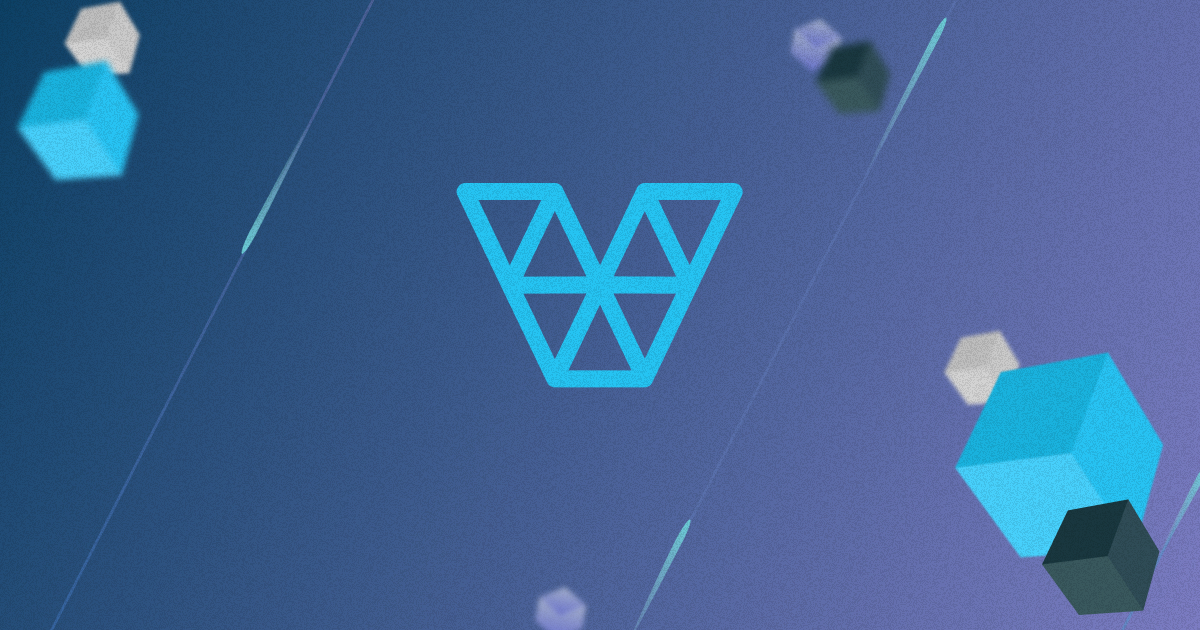
What is Laravel Vapor and why should you use it? In just a few easy steps, deploy your application to AWS with the scalability and simplicity of serverless — without having to manage or provision servers (infrastructure).
Laravel Vapor is an auto-scaling, serverless deployment platform for Laravel, powered by AWS Lambda. It provides multiple features of managing databases, caches, queues, DNS, auto-scaling with simplicity in mind.
Registration and setup
Once you register you automatically get assigned to a “Personal” team. Projects (when created), Networks, Databases, Caches and Domains are associated with the specific team.
Teams are used to organize projects by clients or topics. You should setup billing information under My profile > Billing if you have more then one project.
Connect your AWS account with each team. Choose a team inside Vapor UI and under Dashboard > Team settings > AWS Accounts enter information from AWS account.
Account name can be anything, you can get Access Key ID and Secret Access Key from AWS console (click on account name > Security Credentials > Access keys).
Install Vapor CLI globally using terminal: composer global require laravel/vapor-cli --update-with-dependencies. Also, to make sure you’re running the latest version, execute: composer global update laravel/vapor-cli.
In the terminal, enter vapor to confirm it is successfully installed (if command is not found, add PATH=$(composer global config bin-dir --absolute --quiet):$PATH to your ~/.bashrc).
Application requirements are php 7.3 or higher and laravel/framework 6.0 or higher (check in composer.json file).
We’re going to work only with the default Personal team, but usually you should always check if you have the right team selected – otherwise you will most likely create duplicate of project in another team.
Project initialization and overview
Move into the project folder, if you are not logged in use vapor login command.
Next, execute the vapor init command, enter the region where the project should be placed in, ex. eu-west-1 (choose location closest to your users).
Also, install laravel/vapor-core package when asked. Vapor core contains a service provider that will allow application to run Vapor.
In the Vapor UI you can see a list of your projects for the chosen team. If you open your project you just created, you will see a production environment (act as versions of application). If you want you can add more environments (ex. staging, testing etc).
Under Networks there will be a new network created by Vapor – AWS VPC to secure our resources by isolating them from the outside world. If you click on the created network you can add NAT Gateway or create Load Balancer.
In the root of the project we have a vapor.yml file which contains environments and allows you to configure deployments.
For now we can deploy our application in a production environment, to do so run: vapor deploy production (production represents the environment present in vapor.yml file under environments key).
This will deploy application to the production environment using configuration present in vapor.yml file (read docs for more information).
Deployment steps are shown in the terminal, as well as in the production environment in Laravel Vapor UI for that project.
When you deploy your application to some environment – that environment gets automatically assigned a unique vanity URL (domain), development domain where you can preview your application. Each environment has its own unique vanity domain.
Configure environment and deployment – vapor.yml
When you add a project to Vapor, it creates a vapor.yml file at the root of your project. This file contains the project name, as well options for each environment present in that file. Each environment has its own options, vanity domain and its own features (check out docs). Check the following vapor.yml configuration file.
Commands that run before deployment (build):
The build option (hooks) prepares your application for deployment. Set of commands that you want to run on a local machine (before deployment) to prepare your application for deployment. Build hooks output is shown when we deploy execute:
vapor deploy <environment_name>
Commands that run after deployment (deploy):
The deploy option (hooks) are run after uploading applications to AWS. For example we want to execute a php artisan migrate --force command (use force flag to avoid prompt). Since this is a database migration command and we haven’t created a database using Vapor this command is expected to fail (in step “Running deployment hooks”) when we execute:
vapor deploy <environment_name>
If you need to change the php version, you can do so by changing runtime value (runtime: php-8.1:al2) – runtime max app size is 250MB.
To add new environment, execute: vapor env <new_env_name>
- creates new environment option in vapor.yml file (with name new_env_name)
- creates new environment in list of environments for project in Laravel Vapor UI
Then you deploy it using: vapor deploy new_env_name
Databases
Databases can be created and managed inside Vapor UI or from Vapor-CLI.
To create database go to your Laravel Vapor UI > Resources – Databases and click Create database:
Database – named: vapor-app-database
For a network, choose the one where you want to put all your resources that will connect to your application and environment.
Name that you specify is important (in our case named vapor-app-database) because we will include it later in the vapor.yml file.
Type specifies what kind of database you wish to create (MySQL or PostgreSQL) also you can choose Fixed Size Database or Serverless database. The difference is that if you choose Serverless you don’t have to guess capacity for your application needs, database storage and compute capacity when your application needs it, and shrinks capacity when your app needs less. Choose type depending on your workload.
Backup Retention Window (Days) – it does allow you to restore your database within specified days.
Serverless RDS costs and gives you some different options, described at following image:
Database – serverless type
Choose if your database should be public (free) or private (attaches NAT gateway and jumpbox – both cost).
Lastly you can enable storage encryption if needed.
When we create a database, we get database master user database credentials (save them, if you want to connect to the database from a local machine).
Also there’s no need to include them in the .env file of your project – Vapor does this when you configure the vapor.yml file to use the created database.
Inside vapor.yml file for environment (in our case production), add:
database: vapor-app-database (where vapor-app-database is the name of the database we just created in Vapor UI) as shown.
The last thing you need to do is deploy your project (environment), so execute:
vapor deploy production
Inside Vapor UI > Resources – Databases > vapor-app-database you can manage database users, set alarms (ex. when you need to scale).
To scale your database click on triple dot (…) and click on Scale – this will cause downtime so you may want to put your application in maintenance mode before scaling.
To put your application in maintenance mode, inside Vapor UI > Projects – vapor-app > production, click on triple dot and click on enable maintenance mode.
Since database backups are done automatically, if you want to restore your database to any point in time inside Vapor UI > Resources – Databases > vapor-app-database, click on triple dot (…) and click Restore. The way it works it will create a new database with the content depending on the point in time you specify (within the Backup retention window – image 1.1 – 3 days).
Docker deployments
We could use Vapor pre-built packages for our runtimes, but what if you need some php extension or your application size is greater than 250MB which is max size for Vapor pre-built packages.
Introducing docker runtime which has its limitations for applications up to 10GB in size and gives you ability to install extensions etc. (check out docs for more).
Create Dockerfile at the root of your project with the name of the environment. For a production environment, create a production.Dockerfile at the root of your project.
In the vapor.yml file instead of using runtime like php, for runtime use runtime:docker as shown, where the production.Dockerfile looks like the following.
In order to apply changes execute: vapor deploy production
You can customize Dockerfile as you want, install extensions etc.
You could for example check if FFMPEG library is installed using following command:
vapor tinker --code “echo exec(‘ffmpeg --version’)” production
Vapor Docker images are based on Alpine Linux (read more). We will add FFMPEG library, mysql client, GMP extension and customize php.ini file.
Your production.Dockerfile would look like the this.
Create your php.ini file at the root of your project. Its content may look like the following.
Once you have created both, don’t forget to execute vapor deploy production.
Environment variables
Environment file (.env) that’s inside your project contains values for local development. All environment variables that are in use for specific application environment can be seen inside AWS account:
AWS Lambda > Functions > vapor-<your_app_name>–<your_environment>
> configuration > Environment variables
AWS – vapor-app – production environment – environment variables
<your_app_name> – this app is called “vapor-app”
<your_environment> – deployed “production” environment (specified in vapor.yml)
You can edit those environment variables using Vapor-CLI or inside Vapor UI.
Change environment variables in Vapor UI:
In Vapor UI > Projects – vapor-app > production, click on triple dots (…) on top right corner and choose “Edit Environment Variables”. After changing those values you click Save, and also you need to deploy your application for new environment variables to take effect.
Check out if variables are successfully set after deployment in your AWS account (AWS > Lambda > Functions > vapor-<your_app_name>–<your_environment> > configuration > Environment variables).
Conclusion
You’ve seen how easy it is to deploy Laravel applications using Laravel Vapor. These were just the basics of Laravel Vapor and it has more features than shown.
You can add more teams and privileges per each member, configure domains, execute Tinker commands on environments, configure caches, queues, firewall, monitoring, logging etc.
Hope this blog was helpful for you to learn the basics of Laravel Vapor and how to get started.
Give Kudos by sharing the post!