February 28, 2023 - 10 min
How ChatGPT Can Help You Become a Better Flutter Developer
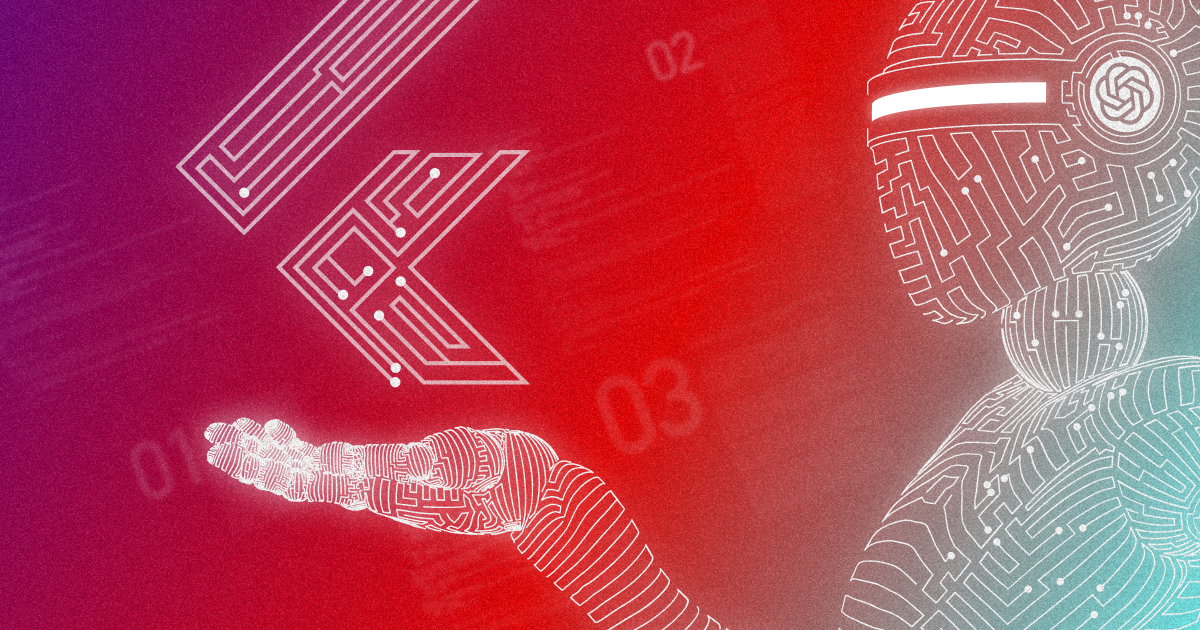
ChatGPT is an advanced machine-learning model that can generate human-like text. Developed by OpenAI, this model has been trained on a wide variety of texts and is capable of producing grammatically correct and contextually relevant sentences. In this blog post, I will show you in what ways ChatGPT can be used as a tool for Flutter developers.
Introduction
As a developer, you spend most of your time googling the answers and searching the internet for possible solutions. What if I told you that there is a new, better way of getting answers?
One where the machine is talking to you like a human would, explaining things in a grammatically correct and contextually relevant way and providing correct answers in a fraction of the time it would take you to find them searching throughout the vast lands of the internet? Meet ChatGPT.
ChatGPT is a state-of-the-art natural language processing model developed by OpenAI. It uses machine-learning techniques to understand and generate human-like text. ChatGPT can be fine-tuned to perform a wide range of natural language tasks such as text generation, text completion, text classification, and more. It’s been trained on a large corpus of text data and can generate coherent, human-like text that often makes it hard to distinguish from text written by a human.
Of course, all this sounds pretty cool, but how exactly can we use ChatGPT as Flutter developers? It’s as easy as typing in a prompt with instructions in natural language, describing what you want to achieve. The AI will follow your exact instructions, so the more detailed you are in the prompt, the better the outcome will meet your needs.
Let’s check out some use cases!
Produce blocks of code using natural language
One of the first ways ChatGPT can help you out is by writing Flutter widgets for us. Here, I simply ask ChatGPT to create a Flutter widget that has an image and some text on top of that image.
Prompt 1
Can you create a Flutter widget that has an image as a background and some text that is centered on top of it?
Answer 1
Yes, here is an example of a BackgroundImageWithCenteredText widget in Flutter that takes an image URL, text, and textStyle as required arguments and renders an image as a background and text on top of it:
import 'package:flutter/material.dart';
class BackgroundImageWithCenteredText extends StatelessWidget {
final String imageUrl;
final String text;
final TextStyle textStyle;
BackgroundImageWithCenteredText({
required this.imageUrl,
required this.text,
required this.textStyle,
});
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
image: DecorationImage(
image: NetworkImage(imageUrl),
fit: BoxFit.cover,
),
),
child: Center(
child: Text(
text,
style: textStyle,
),
),
);
}
}
In this example, a Container widget is used with a BoxDecoration to set the background image. The DecorationImage widget is used with the NetworkImage to set the image and the BoxFit.cover is used to fill the entire container area.
The Center widget is used to center the text on top of the image.
Please note that for this to work you should have the correct dependencies for image_picker and network package in your pubspec.yaml file. You can also use AssetImage instead of NetworkImage if the image is stored locally in your project.
We can clearly see that not only did ChatGPT provide us with an out-of-the-box Flutter widget, but it also described what the widget is doing, which dependencies are required for it to work, and how to interact with it.
Awesome, isn’t it? Let’s see some more examples at work.
Optimize blocks of code
Here I asked ChatGPT to optimize the widget it just generated in Prompt 1. Now, keep in mind, I did not need to specify which widget and how to optimize it, I simply continued our conversation like I would with a person and asked the next question:
Prompt 2
Can you optimize this widget?
Answer 2
Here is one way to optimize this widget:
- Caching the image:
You can use the CachedNetworkImage package which provides a CachedNetworkImage widget that can automatically cache images and show a placeholder until the image is loaded. This can improve the performance of the widget and reduce the amount of data that needs to be loaded over the network.
import 'package:cached_network_image/cached_network_image.dart';
class BackgroundImageWithCenteredText extends StatelessWidget {
final String imageUrl;
final String text;
final TextStyle textStyle;
BackgroundImageWithCenteredText({
required this.imageUrl,
required this.text,
required this.textStyle,
});
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
image: DecorationImage(
image: CachedNetworkImageProvider(imageUrl),
fit: BoxFit.cover,
),
),
child: Center(
child: Text(
text,
style: textStyle,
),
),
);
}
}
- Using the FadeInImage widget:
You can use the FadeInImage widget instead of DecorationImage which automatically shows a fade-in animation when the image is loaded. This can make the image appear more smoothly and improve the overall user experience.
class BackgroundImageWithCenteredText extends StatelessWidget {
final String imageUrl;
final String text;
final TextStyle textStyle;
BackgroundImageWithCenteredText({
required this.imageUrl,
required this.text,
required this.textStyle,
});
@override
Widget build(BuildContext context) {
return Container(
child: Stack(
children: <Widget>[
FadeInImage.memoryNetwork(
placeholder: kTransparentImage,
image: imageUrl,
fit: BoxFit.cover,
),
Center(
child: Text(
text,
style: textStyle,
),
),
],
),
);
}
}
Please note that in order to use the CachedNetworkImage package you should have the correct dependencies in your pubspec.yaml file.
Using either of these two optimizations will help improve the performance of the widget and make it more user-friendly.
Write code documentation or explain code snippets
If you need documentation for a specific piece of code or just an explanation of it, all you have to do is ask ChatGPT to write you some documentation and then provide the piece of code for which you want the documentation to be written.
Prompt 3
Can you write documentation for the next function?
EitherAppFailureOr<AuthResponse> _getUserData() async {
try {
final response = await _authApiClient.getUserInfo();
return Right(response);
} catch (err) {
return Left(Failure.serverError.toAppFailure);
}
}
Answer 3
/// This function makes an API call to get user information, it returns a value of
/// type `EitherAppFailureOr<AuthResponse>` which is a custom type that indicating
/// that the function can return either a success value of type AuthResponse or a failure value
/// of type AppFailure, which is a custom type indicating what type of failure occurred.
EitherAppFailureOr<AuthResponse> _getUserData() async {
try {
final response = await _authApiClient.getUserInfo();
return Right(response);
} catch (err) {
return Left(Failure.serverError.toAppFailure);
}
}
- This function, _getUserData makes an API call to get user information. It starts by attempting to make an API call to get user information, this API call is made by calling the _authApiClient.getUserInfo() method which is an asynchronous function.
- If the API call is successful, the function wraps the response in the Right variant of Either type and returns it, indicating that the function call was successful and the response from the API is included. The response is of type AuthResponse.
- If the API call fails, an error is thrown, and the catch block is executed. The catch block wraps the Failure.serverError.toAppFailure in a Left variant of Either type and returns it. This indicates that the function call failed and an error occurred.
- The EitherAppFailureOr<AuthResponse> is a custom type that indicates that the function can return either a success value of type AuthResponse or a failure value of type AppFailure, which is a custom type indicating what type of failure occurred.
This function can be used in the app to check if the user is authenticated and to get information about the authenticated user.
It is important to note that either AuthResponse or AppFailure should be defined in the codebase and _authApiClient should be initialized before this function can be used.
Detect and fix errors in code
ChatGPT can also find and fix errors in provided code. I’ll purposely provide ChatGPT with a piece of code that has a bug hidden within it and let’s see if it can figure it out.
Prompt 4
Can you detect errors in the code below and fix them?
Future<int> addTwoNumbers(int num1, int num2) async {
// some async work
Future.delayed(Duration(seconds: 2));
return num1+num2;
}
Answer 4
There is an error in the code you provided.
The Future.delayed method returns a new Future that completes after a delay, but it doesn’t do anything with the returned Future, so it is not awaited and doesn’t affect the behavior of the function.
Here is the corrected version:
Future<int> addTwoNumbers(int num1, int num2) async {
await Future.delayed(Duration(seconds: 2));
return num1+num2;
}
In this version, await keyword is added before Future.delayed method, this will make the function wait for the delayed Future to complete before proceeding with the rest of the code.
Therefore, this will correctly add a delay of 2 seconds before returning the result of adding two numbers.
Explaining or generating regular expressions (Regex)
If there is one thing all developers agree on, it’s this. Regex can be super tricky. They’re hard to read and hard to understand, usually, you would use some kind of tool for generating or testing them out.
Well, it just got a lot easier to deal with them. You can simply copy and paste some regular expressions and ask ChatGPT to explain them to you or you can describe what kind of regex you want and ask it to generate it.
Prompt 5
Can you create and explain a regex that matches a valid URL?
Answer 5
^(https?:\/\/)?([\da-z\.-]+)\.([a-z\.]{2,6})([\/\w \.-]*)*\/?$
This regular expression is made up of several parts:
- ^ – Matches the start of the input string.
- (https?:\/\/)? – Matches the optional “http” or “https” protocol at the beginning of the URL. The ? at the end of the group makes the preceding group optional.
- ([\da-z\.-]+) – Matches the domain name. The square brackets [] define a character class, which matches any one character that’s listed inside. The \da-z inside the class matches any digit or lowercase letter. The . and – characters are also matched. The + at the end of the group means that one or more characters in the group must be present.
- \.([a-z\.]{2,6}) – Matches the domain suffix (e.g., “com”, “edu”, “gov”). The . at the start of the group is used to match the dot literally. The {2,6} specifies that the preceding group, which is the [a-z\.] should match between 2 and 6 times.
- ([\/\w \.-]*)* – Matches the path, query string, and/or fragment identifier of the URL. The * at the end of the group means that zero or more characters in the group must be present.
- \/? – Matches the optional trailing slash at the end of the URL.
- $ – Matches the end of the input string
This regex will match URLs with or without HTTP and HTTPS protocol, and it will also match URLs that may have a path, query string and/or fragment identifier. However, it should be noted that this is a complex regex and it may not match all possible URLs, it may not cover all the edge cases, for more complex and thorough URL validation, one should look into using more advanced libraries.
Write test cases in Flutter
Now now, I know nobody really likes or wants to write tests but they are necessary. They allow us to be sure that certain parts and features of the application are working seamlessly and correctly.
Luckily, ChatGPT can also help us out in this area. For example, you can ask it to generate let’s say a unit test and provide the function or a class that you want it to create a test case for or you can describe the class and ask it to generate both class and the unit test for the generated class.
Prompt 6
Create a Counter class that holds value and can increment and decrement that value. Write out a unit test for that class in Flutter.
Answer 6
Here is an example of a Counter class that holds a value and can increment and decrement that value:
class Counter {
int _value;
Counter({int initialValue = 0}) : _value = initialValue;
int get value => _value;
void increment() {
_value++;
}
void decrement() {
_value--;
}
}
And here is an example of a unit test for the Counter class using the flutter_test package:
import 'package:flutter_test/flutter_test.dart';
import 'package:your_project_name/counter.dart';
void main() {
test('Counter increments value', () {
final counter = Counter(initialValue: 0);
counter.increment();
expect(counter.value, 1);
});
test('Counter decrements value', () {
final counter = Counter(initialValue: 0);
counter.decrement();
expect(counter.value, -1);
});
test('Counter increments value by specified amount', () {
final counter = Counter(initialValue: 0);
counter.increment();
counter.increment();
expect(counter.value, 2);
});
test('Counter decrements value by specified amount', () {
final counter = Counter(initialValue: 0);
counter.decrement();
counter.decrement();
expect(counter.value, -2);
});
}
These tests ensure that the increment() and decrement() methods correctly change the value of the Counter class, and that the value getter correctly returns the current value of the counter. It’s also good to test the edge cases and different inputs as well as negative values.
It’s important to test the code to ensure that it behaves as expected and to catch any bugs early on.
Ask general questions about how to do something
Let’s say you never worked with Riverpod in Flutter and you would like an example of how to implement it as a state management solution. All you have to do is ask ChatGPT to provide you with a starting point.
Prompt 7
Can you show me a practical example of how to implement state management using Riverpod into a simple mobile app written in Flutter that has a counter feature?
Answer 7
Sure! Here’s an example of a simple Flutter app that uses the Riverpod package to manage state and has a counter feature:
- First, you’ll need to add the Riverpod package to your pubspec.yaml file:
dependencies:
flutter:
sdk: flutter
riverpod: ^0.14.2
- Next, create a new file called providers.dart in your project and define a Provider for the counter:
import 'package:riverpod/riverpod.dart';
final counterProvider = StateProvider<int>((_) => 0);
This Provider is a simple counter that starts at 0 and can be incremented or decremented.
- In your main.dart file, import providers.dart and wrap the MaterialApp widget in a ProviderScope:
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
import 'providers.dart';
void main() {
runApp(
ProviderScope(
child: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: MyHomePage(),
);
}
}
In addition, this will make the counterProvider available to all of the widgets in the app.
- In your MyHomePage you can use the counterProvider to read and update the counter.
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
final counter = context.watch(counterProvider);
return Scaffold(
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text('Counter: ${counter.state}'),
SizedBox(height: 10),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
FlatButton(
onPressed: () => counter.state--,
child: Text('-'),
),
SizedBox(width: 10),
FlatButton(
onPressed: () => counter.state++,
child: Text('+'),
),
],
),
],
),
),
);
}
}
In this example the watch method is used to access the current state of the counter, the state property of the counter variable is used to get the current value of the counter and the state– and state++ is used to increment or decrement the counter.
- Now you can run your app and see that when you press the “+” and “-” buttons, the counter value is incremented or decremented.
To conclude, this is a very simple example of how to use Riverpod for state management in a Flutter app. Riverpod provides more powerful and flexible state management solutions, but this is a good starting point to understand the basics.
Conclusion
In conclusion, ChatGPT is a powerful tool that can significantly enhance the way we approach Flutter app development. Its ability to understand and respond to natural language instructions, generate code, and assist in debugging and troubleshooting can save us a significant amount of time and effort.
However, it’s important to keep in mind that ChatGPT is not a replacement for developers but rather a productivity booster. As with any machine learning model, the output is only as good as the input.
Furthermore, properly prompting ChatGPT is essential to ensure that the model generates high-quality and relevant outputs. In fact, by taking the time to properly train and prompt the model, developers can ensure that they are getting the most out of ChatGPT and creating high-quality apps faster and more efficiently than ever before.
Give Kudos by sharing the post!